Introduction
In the world of modern web development, delivering high-performance applications that cater to user experience is paramount. React web development has revolutionized how we build user interfaces with its component-based architecture and declarative approach. While client-side rendering is widely popular, server-side rendering (SSR) with React offers unique advantages in terms of SEO, faster initial load times, and improved user experience. This guide delves into best practices for implementing SSR in your React projects while seamlessly integrating it into your development workflows.
If you are a React JS development company or an individual developer looking to enhance your skills, mastering SSR can set you apart by ensuring your applications meet the highest standards of performance and accessibility.
What is Server-Side Rendering (SSR)?
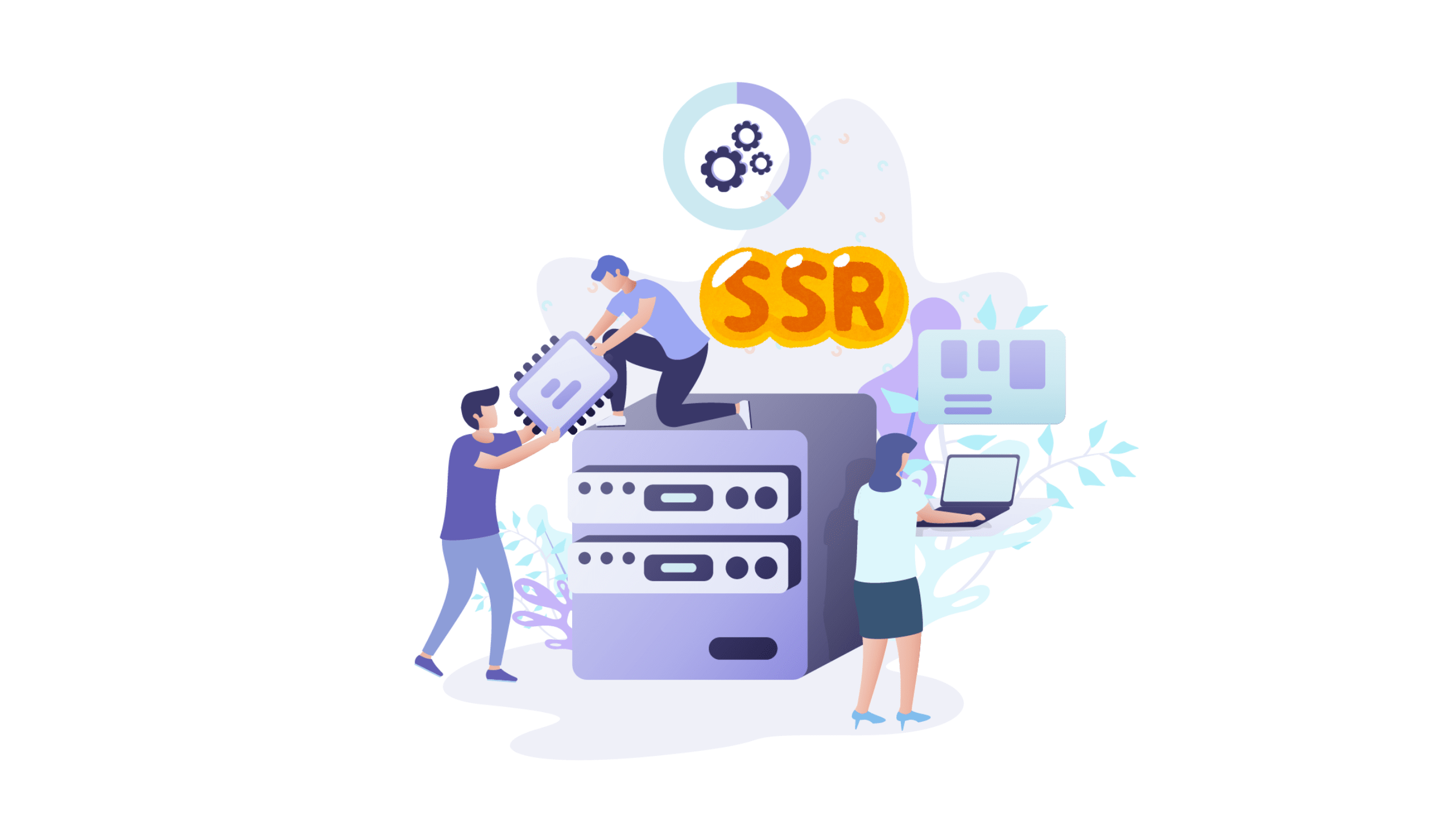
Server-Side Rendering (SSR) is the process of rendering web pages on the server instead of the client. When a user makes a request, the server processes the React components and sends a fully rendered HTML page to the browser. This approach contrasts with client-side rendering (CSR), where JavaScript is responsible for rendering content directly in the browser after the initial page load.
Key Benefits of SSR:
- Improved SEO: SSR provides fully-rendered content to search engine crawlers, enhancing visibility.
- Faster Time to Interactive (TTI): Users receive a pre-rendered page, reducing the perceived load time.
- Better Accessibility: Pre-rendered content ensures compatibility with assistive technologies.
Transform Ideas into ReactJS Solutions!
SDLC CORP delivers cutting-edge, user-friendly ReactJS applications.
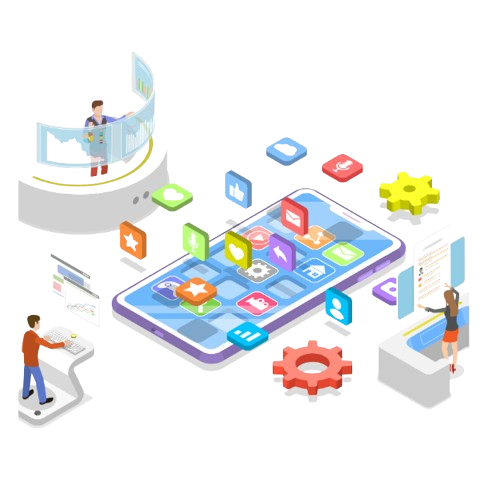
Setting Up Server-Side Rendering in React
1. Initializing the Environment
To implement SSR, you need a Node.js server that can render React components. Most React JS development services recommend using frameworks like Next.js or setting up an Express server manually.
Steps to Set Up SSR:
- Install Required Dependencies:
npm install express react-dom/server react-router-dom
Create a Server File: In your server.js:
import express from ‘express’;
import React from ‘react’;
import { renderToString } from ‘react-dom/server’;
import App from ‘./App’;
const app = express();
app.get(‘*’, (req, res) => {
const appMarkup = renderToString(<App />);
res.send(`
<!DOCTYPE html>
<html>
<head>
<title>React SSR</title>
</head>
<body>
<div id=”root”>${appMarkup}</div>
</body>
</html>
`);
});
app.listen(3000, () => console.log(‘Server is running on http://localhost:3000’));
2. Structuring Your React Application
While working on SSR, maintaining a clean architecture is essential. Split your application into components, services, and utilities to enhance maintainability. React app development teams often use a modular approach to scale SSR applications effectively.
Best Practices for Implementing SSR
1. Optimize Performance
Performance is critical in React website development, especially when rendering on the server. Use these strategies:
- Code Splitting: Leverage dynamic imports to load only the necessary code for each route.
- Caching: Implement server-side caching to reduce repetitive rendering of static content.
- Minify HTML: Use tools to minimize the size of the HTML sent to the browser.
- Code Splitting: Leverage dynamic imports to load only the necessary code for each route.
2. Manage Data Fetching Effectively
Efficient data fetching is a cornerstone of SSR. To ensure the application hydrates correctly on the client side:
- Use libraries like Redux or React Query to handle server-state synchronization.
- Avoid over-fetching data by tailoring API requests to the user’s route and needs.
Also check our latest blog : How to Build a Real-Time Chat App with React
3. Handle Styles and Assets
SSR requires special handling of CSS and other assets. Most React development services integrate solutions like Styled Components or Emotion for seamless server-side style rendering.
- Critical CSS: Extract and inline critical CSS to prevent layout shifts.
- Static Assets: Serve assets like images and fonts through a CDN for faster delivery.
- Critical CSS: Extract and inline critical CSS to prevent layout shifts.
SEO Considerations for SSR Applications
1. Ensure Proper Metadata
When developing for SSR, proper metadata is vital to optimizing search engine visibility. Use libraries like react-helmet to dynamically manage titles and meta tags.
2. Avoid Rendering Errors
Search engine crawlers can struggle with broken rendering. Always test your SSR application for JavaScript errors and incomplete content rendering.
3. Canonical URLs
Integrate canonical tags to prevent duplicate content issues in SSR applications.
Build Modern Web Applications with ReactJS!
SDLC CORP ensures high-quality, responsive apps for all your business needs.
SSR vs. CSR vs. Static Site Generation (SSG)
While SSR has numerous advantages, it’s essential to understand how it compares to other rendering methods:
Server-Side Rendering (SSR):
- Ideal for dynamic content and SEO.
- Requires a Node.js server for real-time rendering.
Client-Side Rendering (CSR):
- Best for applications where interactivity outweighs SEO.
- Reduces server load but increases TTI.
Static Site Generation (SSG):
- Suitable for static websites or blogs.
- Combines pre-rendered HTML with React’s reactivity.
Choosing the right rendering strategy depends on the use case and user requirements. For instance, a React mobile app may not benefit from SSR as much as a content-heavy web application.
Tools and Frameworks for SSR with React
1. Next.js
Next.js is the go-to framework for SSR in React. It simplifies the process of server-side rendering and provides built-in routing, data fetching, and optimization features.
2. Razzle
Razzle offers an out-of-the-box setup for SSR, abstracting webpack and Babel configurations.
3. Custom Express Server
While frameworks like Next.js are convenient, some React JS developers prefer custom solutions using Express for greater flexibility.
Also check our latest blog : Using React Context for Efficient State Management
Challenges in Server-Side Rendering
Despite its benefits, SSR comes with challenges:
- Increased Complexity: Managing server-side logic along with React components adds layers of complexity.
- Hydration Mismatches: Ensuring the server-rendered markup matches the client-side React tree is crucial.
- Performance Overhead: SSR can strain server resources, especially for high-traffic applications.
Hire ReactJS Experts for Your Next Project!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
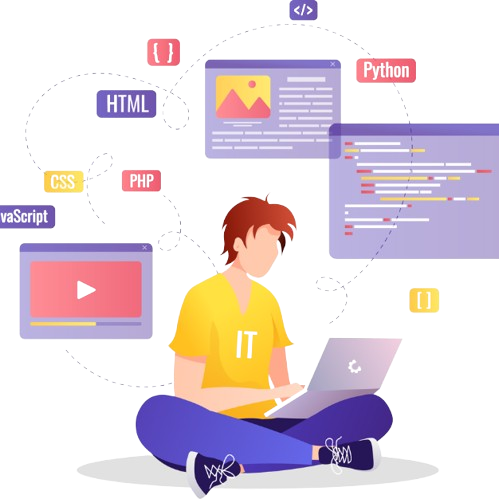
Conclusion
Server-Side Rendering with React is a powerful technique to enhance SEO, improve performance, and deliver a seamless user experience. Whether you’re a React JS development company offering specialized services or a developer exploring new skills, mastering SSR is a valuable addition to your toolkit.
By following the best practices outlined in this guide, you can ensure your applications meet the demands of modern users and search engines alike. Whether you’re focusing on React website development, React app development, or delivering React development services, SSR will help you create fast, responsive, and search-friendly applications. Implement these strategies today to stay ahead in the competitive world of web development.
SDLC CORP ReactJS Development Services Overview
SDLC CORP provides comprehensive react development services to create cutting-edge web and mobile applications that meet your business goals. Our team of skilled ReactJS developers specializes in crafting interactive user interfaces, robust architecture, and scalable solutions tailored to your specific requirements.
With a focus on innovation and efficiency, we utilize the latest ReactJS tools and libraries to ensure fast development, easy maintenance, and optimal performance. From single-page applications (SPAs) to enterprise-grade solutions, SDLC CORP delivers projects that are responsive, feature-rich, and future-ready. Trust us for seamless integration, on-time delivery, and a collaborative development process that aligns with your vision.